Programming with C++
Description
The course is a comprehensive course that provides a solid foundation in the principles of programming using the C++ language. Through a combination of lectures, hands-on exercises, and programming assignments, students will gain a thorough understanding of essential programming concepts and techniques, as well as practical experience in writing C++ code. This course is designed for beginners who have experience in programming in other programming languages.
The course covers:
- Introduction to C++: Evolution of C++, basic syntax, and structure of a C++ program.
- Data Types and Variables: Primitive data types, variables, constants, and type conversions.
- Control Structures: Decision-making statements (if-else, switch), looping statements (for, while, do-while), and nested control structures.
- Functions: Defining and calling functions, function parameters and return values, function prototypes, and recursion. Lambda functions
- Arrays and Strings: Declaring and initializing arrays, accessing array elements, and string manipulation. The use of std::vector and std::string
- Pointers and References: Understanding pointers, pointer arithmetic, dynamic memory allocation, and passing arguments by reference.
- Object-Oriented Programming (OOP): Classes and objects, encapsulation, inheritance, and polymorphism.
- Object life cycle. Copy and move semantics
- Exception Handling: Handling runtime errors and exceptions using try-catch blocks.
- Type-safety. C++ type casts
- Memory-safety. Smart pointers
- Generic Programming. Templates
- Algorithmic Tasks: Introduction to algorithmic problem-solving techniques, including sorting algorithms (e.g., insertion sort), searching algorithms (e.g., linear search, binary search), basic data structures (e.g., linked lists, stacks, queues), implementing graph algorithms.
Upon completion of this course, students will be equipped with the following skills:
- Proficiency in writing, compiling, and debugging C++ programs.
- Understanding of fundamental programming concepts such as variables, control structures, functions, and arrays.
- Ability to apply object-oriented programming principles to design and implement C++ classes and objects.
- Proficient use of pointers and dynamic memory allocation.
- Competence in handling exceptions.
This course aims to provide a comprehensive understanding of C++ programming, orienting to beginners while also offering a solid foundation for more advanced studies or professional endeavors.

is issued on the Luxoft Training form
Objectives
- Develop and enhance your knowledge of C++ programming
Target Audience
- Software Developers
- Junior C++ developers
Prerequisites
- Basic skills in computer science, algorithms and programming paradigms
- An understanding of Object-oriented (OO) Analysis and Design
Roadmap
- C++ Introduction
- Simple data type and Variables
- Expression in C++
- Operators and Function in C++
- Data Types
- Memory access and management
- Object lifecycle in C++
- Inheritance and polymorphism in C++
- Type casting in C++
- Best Practice in C++
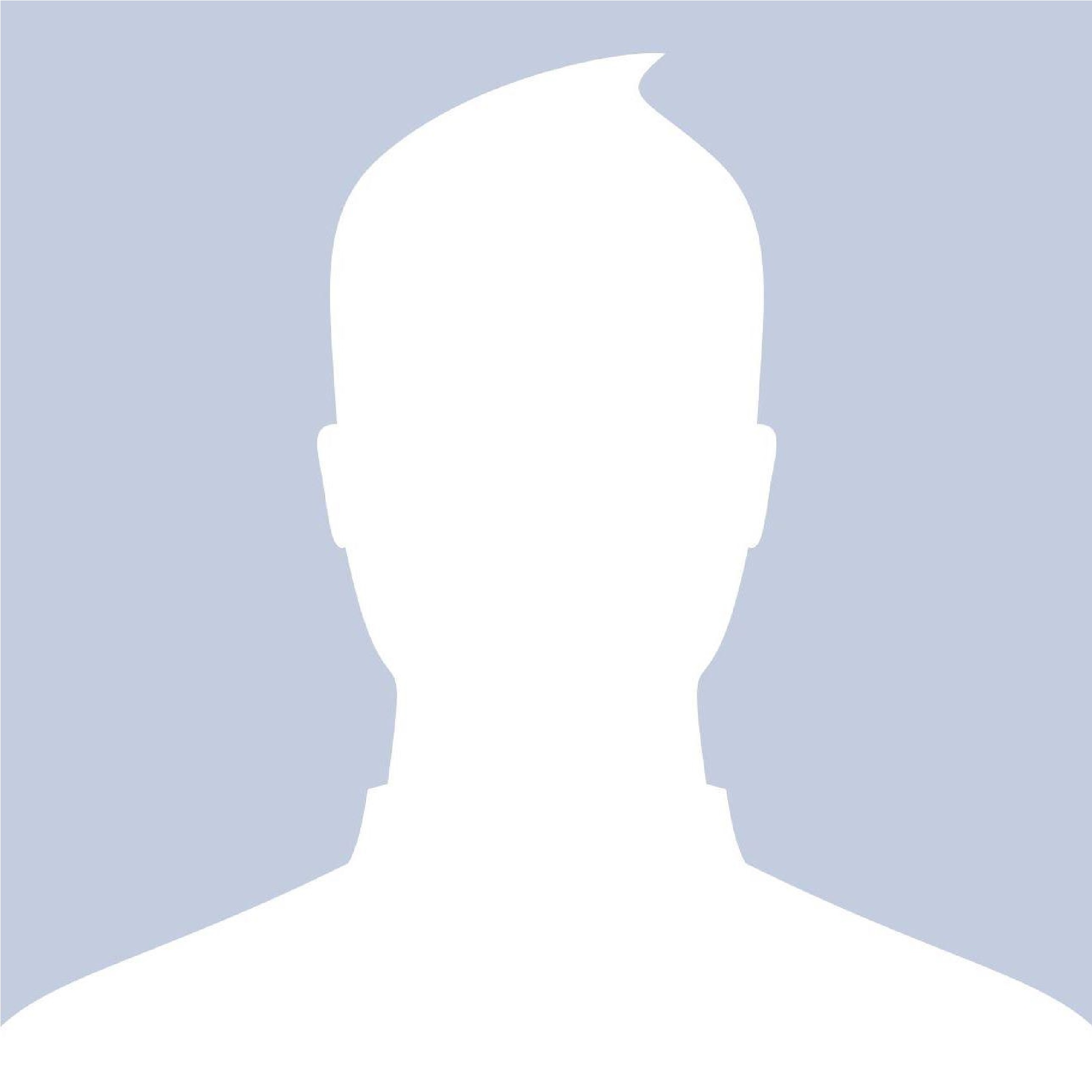